We’ve just open-sourced our application context manager for Laravel
Create multi-context multi-tenant applications with our application context manager package
Not to be confused with Laravel’s new context library, this package can be used to build multi-context multi-tenant applications. Most multi-tenant libraries essentially have a single ‘tenant’ context, so if you need multiple contexts, things can get a bit fiddly. This new package solves that problem.
Let’s look at an example shall we?
Example project
For our example application we’ll have a global user-base that is organised into teams and each team will have multiple projects. This is a fairly common structure in many Software as a Service applications.
It’s not uncommon for multi-tenant applications to have each user-base exist within a tenant context, but for our example application, we want users to be able to join multiple teams, so global user-base it is.
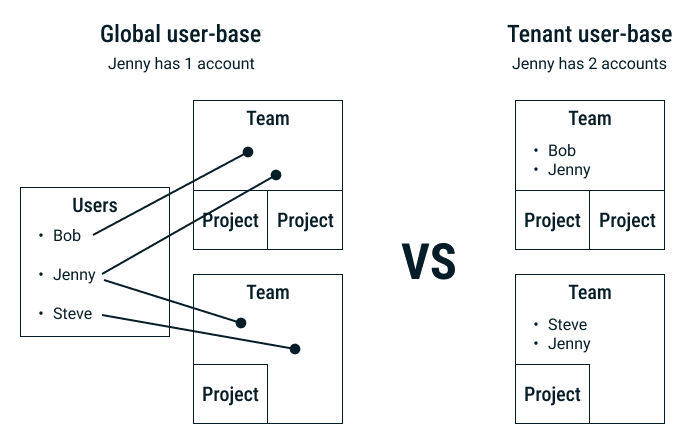
As a SaaS, it’s likely that the team would be the billable entity (i.e. the seat) and certain team members would be granted permission to manage the team. I won’t dive into these implementation details in this example though, but hopefully it provides some additional context.
Installation
To keep this post concise I won’t explain how to start your Laravel project. There are many better resources available for that already, not least the official documentation. let's just assume you already have a Laravel project, with User
, Team
and Project
models, and you’re ready to start implementing our context package.
Installation is a simple composer commend:
composer install honeystone/context
This library has a convenience function, context()
, which as of Laravel 11 clashes with Laravel's own context function. This is not really a problem. You can either import our function:
use function Honestone\Context\context;
Or just use Laravel’s dependency injection container. Throughout this post I will assume you have imported the function and use it accordingly.
The models
Let’s start by configuring our Team
model:
<?php
declare(strict_types=1);
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\BelongsToMany;
use Illuminate\Database\Eloquent\Relations\HasMany;
class Team extends Model
{
protected $fillable = ['name'];
public function members(): BelongsToMany
{
return $this->belongsToMany(User::class);
}
public function projects(): HasMany
{
return $this->hasMany(Project::class);
}
}
A team has a name, members and projects. Within our application, only members of a team will be able to access the team or its projects.
Okay, so let’s look at our Project
:
<?php
declare(strict_types=1);
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\BelongsTo;
class Project extends Model
{
protected $fillable = ['name'];
public function team(): BelongsTo
{
return $this->belongsTo(Team::class);
}
}
A project has a name and belongs to a team.
Determining the context
When someone accesses our application, we need to determine which team and project they are working within. To keep things simple, let’s handle this with route parameters. We’ll also assume that only authenticated users can access the application.
Neither team nor project context: app.mysaas.dev
Only team context: app.mysaas.dev/my-team
Team and project context: app.mysaas.dev/my-team/my-project
Our routes will look something like this:
Route::middleware('auth')->group(function () {
Route::get('/', DashboardController::class);
Route::middleware(AppContextMiddleware::Class)->group(function () {
Route::get('/{team}', TeamController::class);
Route::get('/{team}/{project}', ProjectController::class);
});
});
This is a very inflexible approach, given the potential for namespace clashes, but it keeps the example concise. In a real world application you’ll want to handle this a little differently, perhaps anothersaas.dev/teams/my-team/projects/my-project
or my-team.anothersas.dev/projects/my-project
.
We should look at our AppContextMiddleware
first. This middleware initialises the team context and, if set, the project context:
<?php
declare(strict_types=1);
namespace App\Http\Middleware;
use function Honestone\Context\context;
class TeamContextMiddleware
{
public function handle(Request $request, Closure $next): mixed
{
//pull the team parameter from the route
$teamId = $request->route('team');
$request->route()->forgetParameter('team');
$projectId = null;
//if there's a project, pull that too
if ($request->route()->hasParamater('project')) {
$projectId = $request->route('project');
$request->route()->forgetParameter('project');
}
//initialise the context
context()->initialize(new AppResolver($teamId, $projectId));
}
}
To start with we grab the team id from the route and then forget the route parameter. We don’t need the parameter reaching our controllers once it’s in the context. If a project id is set, we pull that too. We then initialise the context using our AppResolver
passing our team id and our project id (or null
):
<?php
declare(strict_types=1);
namespace App\Context\Resolvers;
use App\Models\Team;
use Honeystone\Context\ContextResolver;
use Honeystone\Context\Contracts\DefinesContext;
use function Honestone\Context\context;
class AppResolver extends ContextResolver
{
public function __construct(
private readonly int $teamId,
private readonly ?int $projectId = null,
) {}
public function define(DefinesContext $definition): void
{
$definition
->require('team', Team::class)
->accept('project', Project::class);
}
public function resolveTeam(): ?Team
{
return Team::with('members')->find($this->teamId);
}
public function resolveProject(): ?Project
{
return $this->projectId ?: Project::with('team')->find($this->projectId);
}
public function checkTeam(DefinesContext $definition, Team $team): bool
{
return $team->members->find(context()->auth()->getUser()) !== null;
}
public function checkProject(DefinesContext $definition, ?Project $project): bool
{
return $project === null || $project->team->id === $this->teamId;
}
public function deserialize(array $data): self
{
return new static($data['team'], $data['project']);
}
}
A little bit more going on here.
The define()
method is responsible for defining the context being resolved. The team is required and must be a Team
model, and the project is accepted (i.e. optional) and must be a Project
model (or null
).
resolveTeam()
will be called internally on initialisation. It returns the Team
or null
. In the event of a null
response, the CouldNotResolveRequiredContextException
will be thrown by the ContextInitializer
.
resolveProject()
will also be called internally on initialisation. It returns the Project
or null
. In this case a null
response will not result in an exception as the project is not required by the definition.
After resolving the team and project, the ContextInitializer
will call the optional checkTeam()
and checkProject()
methods. These methods carry out integrity checks. For checkTeam()
we ensure that the authenticated user is a member of the team, and for checkProject()
we check that the project belongs to the team.
Finally, every resolver needs a deserialization()
method. This method is used to reinstate a serialised context. Most notably this happens when the context is used in a queued job.
Now that our application context is set, we should use it.
Accessing the context
As usual, we’ll keep it simple, if a little contrived. When viewing the team we want to see a list of projects. We could build our TeamController
to handle this requirements like this:
<?php
declare(strict_types=1);
namespace App\Http\Controllers;
use Illuminate\View\View;
use function compact;
use function Honestone\Context\context;
use function view;
class TeamController
{
public function __invoke(Request $request): View
{
$projects = context('team')->projects;
return view('team', compact('projects'));
}
}
Easy enough. The projects belonging to the current team context are passed to our view. Imagine we now need to query projects for a more specialised view. We could do this:
<?php
declare(strict_types=1);
namespace App\Http\Controllers;
use Illuminate\View\View;
use function compact;
use function Honestone\Context\context;
use function view;
class ProjectQueryController
{
public function __invoke(Request $request, string $query): View
{
$projects = Project::where('team_id', context('team')->id)
->where('name', 'like', "%$query%")
->get();
return view('queried-projects', compact('projects'));
}
}
It’s getting a little fiddly now, and it’s far too easy to accidentally forget to ‘scope’ the query by team. We can solve this using the BelongsToContext
trait on our Project
model:
<?php
declare(strict_types=1);
namespace App\Models;
use Honeystone\Context\Models\Concerns\BelongsToContext;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\BelongsTo;
class Project extends Model
{
use BelongsToContext;
protected static array $context = ['team'];
protected $fillable = ['name'];
public function team(): BelongsTo
{
return $this->belongsTo(Team::class);
}
}
All project queries will now be scooped by the team context and the current Team
model will be automatically injected into new Project
models.
Let’s simplify that controller:
<?php
declare(strict_types=1);
namespace App\Http\Controllers;
use Illuminate\View\View;
use function compact;
use function view;
class ProjectQueryController
{
public function __invoke(Request $request, string $query): View
{
$projects = Project::where('name', 'like', "%$query%")->get();
return view('queried-projects', compact('projects'));
}
}
That’s all folks
From here onwards, you’re just building your application. The context is easily at hand, your queries are scoped and queued jobs will automagically have access to the same context from which they were dispatched.
Not all context related problems are solved though. You’ll probably want to create some validation macros to give your validation rules a little context, and don’t forget manual queries will not have the context automatically applied.
If you’re planning to use this package in your next project, we’d love to hear from you. Feedback and contribution is always welcome.
You can checkout the GitHub repository for additional documentation. If you find our package useful, please drop a star.
Until next time..
Share this post